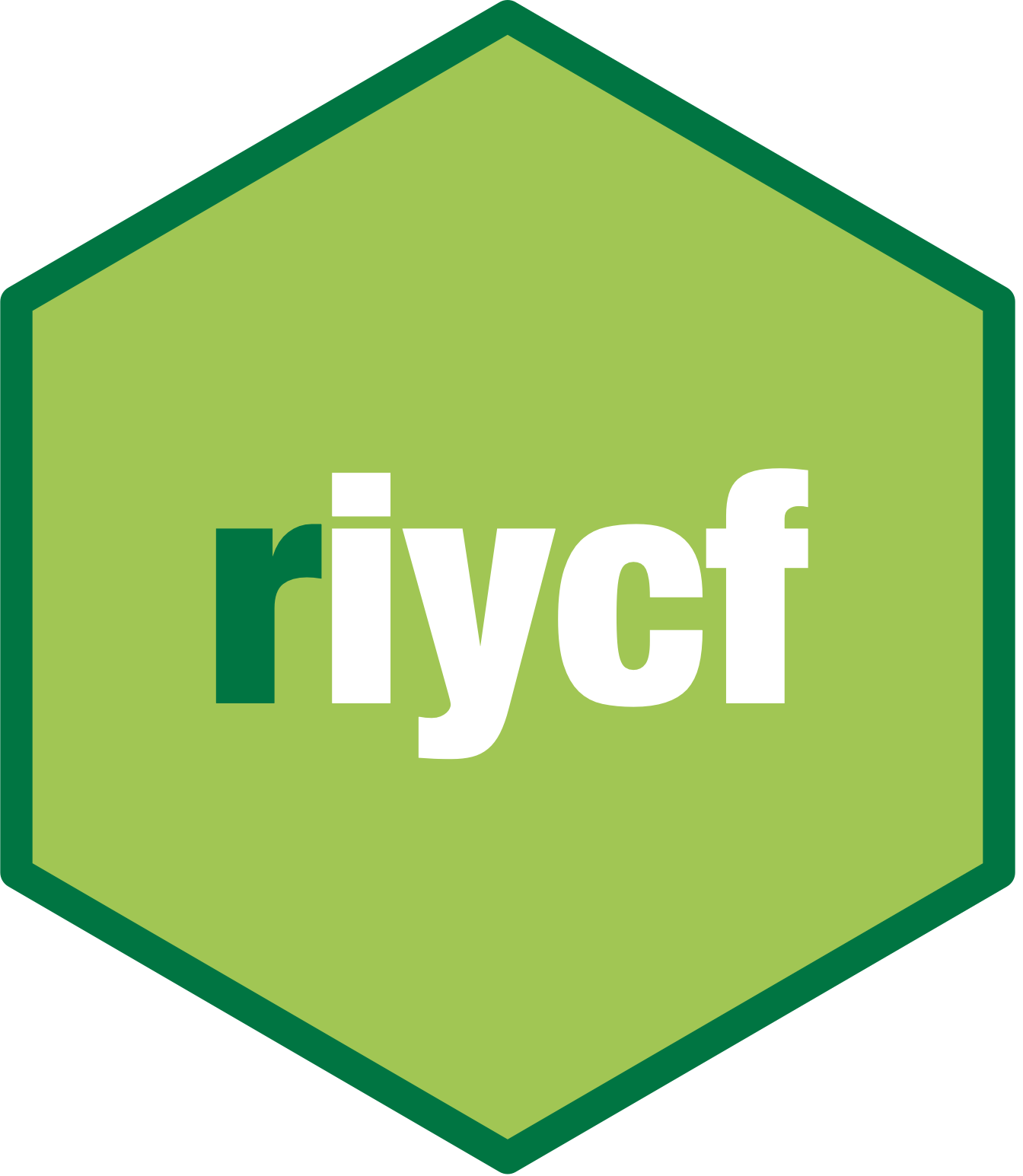
Minimum Diet Related Indicators
Nicholus Tint Zaw
Source:vignettes/minimum_diet.Rmd
minimum_diet.Rmd
Introduction
This article will mainly demonstrate how the riycf
package’s function can apply to calculating minimum diet-related
indicators. According to the new WHO 2021 guideline, there are four
types of complementary feeding practice indicators related to minimum
diet requirements;
- Minimum Dietary Diversity 6-23 Months (MDD),
- Minimum Meal Frequency 6-23 Months (MMF),
- Minimum Milk Feeding Frequency For Non-Breastfed Children 6-23 Months (MMFF) and
- Minimum Acceptable Diet.
Dataset Set-up
Firstly, load the required libraries for data analysis work. We will
use riycf
package for the IYCF indicator generation
work.
For this guideline demonstration, we will use the sample dataset provided by the CARE Myanmar team on IYCF modules. A detailed description of the variable’s name and variable labels are mentioned in the documentation. Use the following syntax to check for the dataset description.
?iycfData
df <- iycfData
head(df[1:5])
#> csex calc_age_months child_bf child_eibf child_eibf_hrs
#> 1 1 5 1 0 NA
#> 2 1 4 1 0 NA
#> 3 1 2 1 0 NA
#> 4 1 0 1 0 NA
#> 5 1 2 1 0 NA
#> 6 1 2 1 0 NA
This dataset contains 359 observations and 46 variables, and all variables were organized based on the WHO IYCF sample questionnaire. We tried to mention explicitly matching function inputs parameters and question numbers from the WHO IYCF sample question. If your dataset did not have the same variables as the WHO sample one, please apply the most relevant ones in the respective input parameter.
Minimum Diet Related Indicators
Minimum Dietary Diversity 6-23 Months (MDD)
Calculation of MDD included three main steps: (1) identification of food groups that were consumed by the children 6-23 months of age in the previous days, (2) calculation of the total number of food groups (out of total 8 food groups) consumed in the previous day and (3) identification of children who meet the minimum requirement of food groups (at least 5 food groups) in their previous day’s consumption.
Individual food group consumption: dummy variables
As the first step of MDD, we will now identify the food group the
children consumed the previous day. We will use the
get_dummy
function to generate the dummy variable for each
8 food groups:
- breast milk;
- grains, white/pale starchy roots, tubers and plantains;
- beans, peas, lentils, nuts and seeds;
- dairy products (milk, infant formula, yogurt, cheese);
- flesh foods (meat, fish, poultry, organ meats);
- eggs;
- vitamin A-rich fruits and vegetables; and
- other fruits and vegetables.
Please note that the input parameter of the get_dummy
function is the list
, not vector
. We need to
prepare the list of variables for the food items we would like to
include in each food group dummy variable calculation. In this article,
I used the same variables applied in 2021 WHO IYCF indicators guideline.
The detailed description of the input variables used for each food group
calculation can be checked using the following syntax in the sample
dataset description file.
?iycfData
For the breastfeeding dummy variable, the guideline-recommended to
using the Question 4
: breastfed yesterday during the day
or at night.
For grains-related food groups, including grains, white/pale starchy
roots, tubers and plantains, we used Question 7B
and
Question 7D
from the WHO IYCF sample questionnaires.
For pulses food groups which include beans, peas, lentils, nuts, and
seeds, we only need to use one variable, Question 7N
.
In the dairy products food group, the following foods items are
included; milk, infant formula, yogurt, and cheese) and the following
questions from WHO guidelines provide that information;
Question 6B
, Question 6C
,
Question 6D
, Question 7A
, and
Question 7O
.
dairy_list <- list(df$child_bms, df$child_milk, df$child_mproduct, df$child_yogurt, df$child_yogurt)
df$dairy <- get_dummy(var_list = dairy_list)
The information about flesh foods, meat, fish, poultry, and organ
meats, is available from the following four questions from the
guideline; Question 7I
, Question 7J
,
Question 7K
, and Question 7M
.
meat_list <- list(df$child_organ, df$child_insects, df$child_beef, df$child_fish)
df$meat <- get_dummy(var_list = meat_list)
Only one variable requires for the Eggs food group, which is
Question 7L
.
We will use the following questions from the guideline for
calculating vitamin A-rich fruits and vegetables;
Question 7C
, Question 7E
, and
Question 7G
.
vita_fruveg_list <- list(df$child_pumpkin, df$child_leafyveg, df$child_mango)
df$vita_fruveg <- get_dummy(var_list = vita_fruveg_list)
We used the following two variables for other fruits and vegetable
food groups; Question 7F
and Question 7H
.
Food groups score claculation
Then, we can now calculate the total food group score the children consumed on the previous day. We will use all the individual food group variables in this calculation.
df$food_score <- get_foodscore(breastmilk = df$breastmilk,
grains = df$grains,
pulses = df$pulses,
dairy = df$dairy,
meat = df$meat,
eggs = df$eggs,
vita_fruveg = df$vita_fruveg,
oth_fruveg = df$oth_fruveg)
table(df$food_score)
#>
#> 0 1 2 3 4 5 6 7 8
#> 3 30 32 43 28 18 3 4 1
summary(df$food_score)
#> Min. 1st Qu. Median Mean 3rd Qu. Max. NA's
#> 0.000 2.000 3.000 2.957 4.000 8.000 197
Minimum Meal Frequency 6-23 Months (MMF)
Unlike MDD, the MMF requires more complex criteria, and those depend on the children breastfeeding status and the age range. We need four essential information to calculate this indicator; child age, breastfeeding status, meal frequency, and milk (beside breastmilk) feeding frequency.
Breastfed child
The minimum meal frequency for breastfeeding varies by the child’s age. It only requires at least two meals for 6-8 months old children, but at least three times for children of 9-23 months old. Below are the sample syntax using three functions to calculate the minimum meal frequency for breastfeeding children.
-
get_mmf_bf_6to8
- to calculate the minimum meal frequency for 6-8 months old breastfed children -
get_mmf_bf_9to23
- to calculate the minimum meal frequency for 6-8 months old breastfed children -
get_mmf_bf
- to calculate the minimum meal frequency for 6-8 months old breastfed children
# BF 6-8 months
df$mmfbf_6to8 <- get_mmf_bf_6to8(q4 = df$child_bfyest,
q8 = df$child_food_freq,
age = df$calc_age_months)
table(df$mmfbf_6to8)
#>
#> 0 1
#> 7 14
# BF 9-23 months
df$mmfbf_9to23 <- get_mmf_bf_9to23(q4 = df$child_bfyest,
q8 = df$child_food_freq,
age = df$calc_age_months)
table(df$mmfbf_9to23)
#>
#> 0 1
#> 24 28
# Overall BF
df$mmf_bf <- get_mmf_bf(mmfbf_6to8 = df$mmfbf_6to8,
mmfbf_9to23 = df$mmfbf_9to23)
table(df$mmf_bf)
#>
#> 0 1
#> 31 42
Non-Breastfed child
In MMF calculation for non-breastfeeding children, we need to
consider the number of milk feeds and the meal frequency. To get that,
use the get_nonbf_frq
function to calculate the milk &
meal feeding frequency using the following questions;
-
Question 6Bnum
: infant formula feeding frequency, -
Question 6Cnum
: animal milk feeding frequency, -
Question 6Dnum
: milk-product liquid feeding frequency and -
Question 8
: meal frequency.
df$nonbf_frq <- get_nonbf_frq(q6bnum = df$child_bms_freq,
q6cnum = df$child_milk_freq,
q6dnum = df$child_mproduct_freq,
q8 = df$child_food_freq)
table(df$nonbf_frq)
#> < table of extent 0 >
sum(df$nonbf_frq)
#> [1] NA
Then, calculate the minimum meal frequency for the 6-23 months of
non-breastfeeding children using that milk & meal frequency
information calculate above. Whoever gets at least 4-times milk and meal
frequency in the previous are identified as the children who get minimum
meal frequency for non-breastfed children. Use the
get_mmf_nonbf
function to calculate this indicator as the
sample syntax provided below.
df$mmf_nonbf <- get_mmf_nonbf(q4 = df$child_bfyest,
q8 = df$child_food_freq,
nonbf_frq = df$nonbf_frq,
age = df$calc_age_months)
table(df$mmf_nonbf)
#> < table of extent 0 >
Calculation of MMF
After calculating minimum meal frequency for breastfed and
non-breastfed children separately, use the get_mmf
function
to consolidate as one overall minimum meal frequency indicator for
reporting. We also frequently report this indicator in the aggregated
figure by breastfeeding status and age range (for breastfed children).
Therefore, all the immediate variables we created along the path to
calculate this overall MMF indicator are also helpful for reporting
work.
Minimum Milk Feeding Frequency For Non-Breastfed Children 6-23 Months (MMFF)
This is the minimum milk feeding frequency indicator for
non-breastfeeding children. It requires two-stage of calculations; (1)
calculating the milk feeding frequency score and (2) calculating the
MMFF indicator. From our package, we can use the
get_milk_frq
function to get this score, and the following
input information is required for this score;
-
Question 6Bnum
: infant formula feeding frequency, -
Question 6Cnum
: animal milk feeding frequency, -
Question 6Dnum
: milk product liquid feeding frequency, and -
Question 7Anum
: the milk-product solid food feeding frequency.
df$milk_frq <- get_milk_frq(q6bnum = df$child_bms_freq,
q6cnum = df$child_milk_freq,
q6dnum = df$child_mproduct_freq,
q7anum = df$child_yogurt)
table(df$milk_frq)
#> < table of extent 0 >
sum(df$milk_frq)
#> [1] NA
Whoever 6-23 months old non-breastfed children who get at least two
milk feeds from the previous day are considered minimally milk-fed
children. Use the get_mmff
function to calculate this
indicator, and it only requires two input parameters; age and milk
feeding frequency score.
Minimum Acceptable Diet
Using all the information calculated before in this article; minimum dietary diversity, minimum meal frequency, and minimum milk frequency for non-breastfeed children. Whoever 6-23 months old children who meet the following condition are identified as children who get the minimum acceptable diet; + get a minimum dietary diversity, + get minimum meal frequency (for both breastfed or non-breastfed), and + get breastfed or get minimum milk feeding frequency.
We will use the get_mad
function to calculate this MAD
indicator, and sample syntax is provided below.